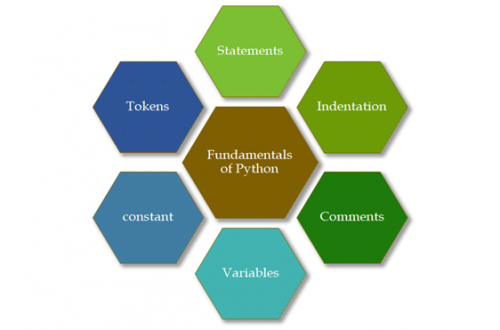
Python Fundamentals
English | Size: 1.46 GB
Category: CBTS
Get started with Python in a practical, hands-on way! Learn types in Python like basic data types, tuples, and dictionaries. Understand control flow in Python programs with loops, boolean statements, if statements, and return statements. Then you’ll make concise, reusable functions, learn how to work with files on your filesystem, and write programs that interact with APIs!
This course and others like it are available as part of our Frontend Masters video subscription.
Table of ContentsIntroduction
Introduction
00:00:00 – 00:04:01
Introduction
Nina Zakharenko introduces the course repository, the course website, and how audience members can connect with her via social media. – https://learnpython.netlify.com/01-introduction/ https://github.com/nnja/python
System Requirements
00:04:02 – 00:10:16
System Requirements
Nina goes over the mandatory prerequisites to be installed before beginning the course.
Opening the Project and Python File
00:10:17 – 00:13:37
Opening the Project and Python File
Nina demonstrates how to set up a basic file structure, create a python file, and a few handy keyboard shortcuts for VSCode.
Starting the Python REPL
00:13:38 – 00:15:54
Starting the Python REPL
Nina demonstrates how to start Python’s read evaluate print loop.
Using the Python REPL
00:15:55 – 00:19:33
Using the Python REPL
Nina introduces the basics of how to use the Python REPL.
Common REPL Mistakes
00:19:34 – 00:23:07
Common REPL Mistakes
Nina demonstrates an error that may occur when reassigning reserved variables, how to write comments, and the basics of how to debug in the REPL
Why Python
00:23:08 – 00:29:02
Why Python
Nina introduces the context of Python, and resources to find best practice principles.
Code Sample
00:29:03 – 00:32:42
Code Sample
Nina walks through a piece of code intended to expose students to the language.
Data Types
Variables
00:32:43 – 00:35:52
Variables
Nina introduces variables by speaking to naming conventions, a few “gotchas”, as well as NoneType and reassigning variables.
Numbers
00:35:53 – 00:38:29
Numbers
Nina demonstrates how to assign integers, floats, complex numbers. The methods associated with types are also addressed as well as basic mathematical operations.
Strings
00:38:30 – 00:45:19
Strings
Nina introduces best practices for strings, string concatenation, long strings, and f strings
Practice: Data Types
00:45:20 – 00:52:22
Practice: Data Types
Students are instructed to display the type of several variables in their REPL. Nina live codes the solution to the exercise.
Functions
Functions
00:52:23 – 01:03:46
Functions
Nina introduces why functions are useful, demonstrates the syntax for a function, and gives examples to demonstrate what returning a value means.
Function Arguments
01:03:47 – 01:13:07
Function Arguments
Nina demonstrates how to add inputs to functions.
Empty Default Lists
01:13:08 – 01:17:53
Empty Default Lists
Nina warns the audience against using empty default lists as a default parameter, due to the mutability rules that will be discussed later in the course.
Function Scope
01:17:54 – 01:25:37
Function Scope
Nina covers the basics of the way that access to variables is handled.
Practice: Functions
01:25:38 – 01:34:13
Practice: Functions
Nina live codes the solution to the exercise.
Lists
Lists
01:34:14 – 01:46:10
Lists
Nina introduces how to instantiate lists.
Changing the Order of a List
01:46:11 – 01:52:15
Changing the Order of a List
Nina introduces methods to change the order of lists, given their contents, then goes on to view all the methods available to lists, and how to see what a particular method does from the REPL.
Adding Items to a List
01:52:16 – 01:55:08
Adding Items to a List
Nina introduces the append, insert, and extend methods built into python.
List Lookups
01:55:09 – 02:00:18
List Lookups
Nina demonstrates how to determine if specific objects are in a list, the index of the first time the object is found in a list, and the number of times an object appears in a list.
Removing Items from a List
02:00:19 – 02:03:07
Removing Items from a List
Nina introduces the remove, pop, and push
List Review
02:03:08 – 02:06:31
List Review
Nina reviews the methods learned in the previous sections.
Tuples
Tuples
02:06:32 – 02:12:27
Tuples
Nina explains why a tuple might be useful, and how to instantiate them.
Unpacking Tuples
02:12:28 – 02:16:33
Unpacking Tuples
Nina demonstrates how to assign variables to a tuple’s values for use outside of the tuple.
Tuples Review
02:16:34 – 02:18:19
Tuples Review
Nina reviews what was covered in the previous sections.
Sets
Sets
02:18:20 – 02:26:36
Sets
Nina introduces what a set is, and why it’s a powerful data structure.
Adding, Removing, & Updating
02:26:37 – 02:30:08
Adding, Removing, & Updating
Nina demonstrates how to add elements, and to remove or replace elements.
Combining, Comparing, & Contrasting
02:30:09 – 02:36:59
Combining, Comparing, & Contrasting
Nina demonstrates how to create a set with elements from two sets, how to find the similarities between sets, and how to find how two sets differ. Frozen sets are also briefly covered.
Set Review
02:37:00 – 02:40:26
Set Review
Nina reviews what was covered in the previous sections.
Dictionaries
Dictionaries
02:40:27 – 02:49:01
Dictionaries
Nina introduces how dictionaries are composed in Python, then goes on to demonstrate how to access values, and set default values if the value specified is null.
Adding, Removing, & Accessing Keys or Values
02:49:02 – 02:55:46
Adding, Removing, & Accessing Keys or Values
Nina demonstrates how to add items, remove items, and access either the keys or values independently from each other using built-in functions.
Dictionaries Review
02:55:47 – 02:58:02
Dictionaries Review
Nina reviews what was covered in the previous sections.
Mutability Review
02:58:03 – 02:59:13
Mutability Review
Nina briefly reviews what mutability is, and covers reinforced which data structures in Python are mutable.
Practice: Advanced Data Types
02:59:14 – 03:11:26
Practice: Advanced Data Types
Students are instructed to try typing out their own lists, dictionaries, tuples, and sets using the instructions on the course website. Nina live codes the exercise with students.
Boolean Logic
Booleans
03:11:27 – 03:15:40
Booleans
Nina introduces truthyness and falseyness in Python.
Comparisons
03:15:41 – 03:21:51
Comparisons
Nina introduces equality and identity.
and, or, & not
03:21:52 – 03:26:04
and, or, & not
Nina introduces boolean logic built in to Python.
Practice: Boolean Logic
03:26:05 – 03:30:02
Practice: Boolean Logic
Nina live code the exercise with students.
Looping & Control Flow
Looping Over Lists
03:30:03 – 03:35:05
Looping Over Lists
Nina introduces “for in” syntax as well as how to utilize the range keyword.
Looping Over Dictionaries
03:35:06 – 03:40:05
Looping Over Dictionaries
Nina demonstrates how to loop over dictionaries.
Control Flow
03:40:06 – 03:43:36
Control Flow
Nina demonstrates how to utilize control flow logic statements.
while & Control Statements
03:43:37 – 03:53:23
while & Control Statements
Nina demonstrates how to implement while loops, continue, and break.
Real-World
Python Files & Modules
03:53:24 – 03:58:25
Python Files & Modules
Nina demonstrates how to start a file in Python, and enable syntax highlighting.
Debugging Strategies in Python
03:58:26 – 04:00:47
Debugging Strategies in Python
Nina demonstrates how to debug a python file using print statements.
Importing Modules, & the main Method
04:00:48 – 04:08:47
Importing Modules, & the main Method
Nina demonstrates how to import files into another file, how to create a module using dunder name, and briefly gives an example of how to include an error message in your log.
External Modules with PIP
04:08:48 – 04:10:21
External Modules with PIP
Nina introduces PIP, which is a tool that allows users to install external libraries.
APIs Overview
04:10:22 – 04:14:14
APIs Overview
Nina explains what APIs are, responses, status codes, and authentication.
requests Library
04:14:15 – 04:19:12
requests Library
Nina demonstrates how to utilize a library that specializes in helping with API requests to get pictures of dogs from an API.
App Solution 1: Fetching the Github API
04:19:13 – 04:24:44
App Solution 1: Fetching the Github API
Students are instructed to utilize the Github API to query for popular courses and sort them by the number of stars that they have. Nina begins live-coding the solution by showing how to begin using Github API.
App Solution 2: Searching the Github API
04:24:45 – 04:35:59
App Solution 2: Searching the Github API
Nina demonstrates how to apply search parameters to the Github API query, and starts to divide the code into separate functions.
App Solution 3: Error Handling
04:36:00 – 04:40:03
App Solution 3: Error Handling
Nina demonstrates how to add error handling to the code so that the user is given a useful error should the API not return the correct response.
Wrap Up
Wrapping Up
04:40:04 – 04:40:50
Wrapping Up
Nina gives some next steps to programmers who want to learn more about Python.
DOWNLOAD:
https://rapidgator.net/file/09c9ea61e8e1b21c3cc512cc1210f569/Python_Fundamentals.part1.rar.html
https://rapidgator.net/file/709f8b9867bea05a7cf91da466a7ca54/Python_Fundamentals.part2.rar.html
https://rapidgator.net/file/169575f51c5675b9c7882705ed25a134/Python_Fundamentals.part3.rar.html
https://nitroflare.com/view/D0A52093FB32AA9/Python_Fundamentals.part1.rar
https://nitroflare.com/view/8E96BC25061A9E2/Python_Fundamentals.part2.rar
https://nitroflare.com/view/01410A23FDDB138/Python_Fundamentals.part3.rar
Leave a Reply